What Is Windows Application Driver (WinAppDriver)?
WinAppDriver is a service to support Selenium-like UI Test Automation on Windows Applications. This service supports testing Universal Windows Platform (UWP), Windows Forms (WinForms), Windows Presentation Foundation (WPF), and Classic Windows apps on Windows 10 PC.
How to Install & Run WinAppDriver?

Open WinAppDriver.exe from the installation directory (C:\Program Files (x86)\Windows Application Driver)
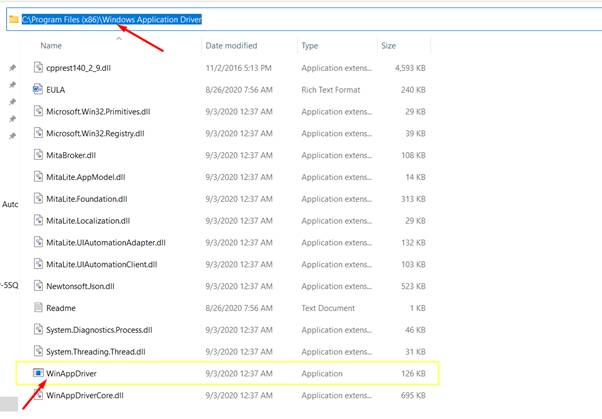
After That, WinAppDriver will be running on the test machine listening to requests on the default IP address and port (127.0.0.1:4723).

Now you are ready to run any of your Tests Scripts.
Read Also:- Most Popular Tools for Automation Testing
Required Packages in Project’s Solutions
We need to add packages ‘Microsoft.WinAppDriver.Appium.WebDriver’ in the current solution from the Nugget as below I have attached the screenshot of the packages.

How to Inspect Element on Windows Application?
First, we need to Download the Win SDK file from https://developer.microsoft.com/en-us/windows/downloads/windows-sdk/
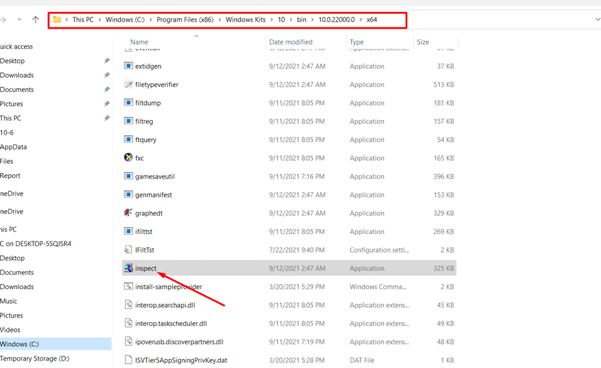
By using ‘inspect.exe’ we can inspect the element present in the windows application

FOR EXAMPLE, I HAVE WRITTEN A DEMO SCRIPT TO PERFORM ACTION ON THE OUTLOOK APP:
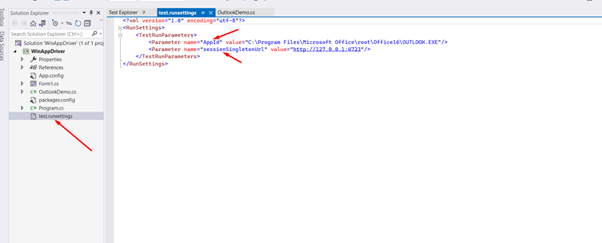
<?xml version="1.0" encoding="utf-8"?>
<Parameter name="AppId" value="C:\Program Files\Microsoft Office\root\Office16\OUTLOOK.EXE"/>
<Parameter name="sessionSingletonUrl" value="http://127.0.0.1:4723"/> //When open WinAppDriver You will get this value.

using OpenQA.Selenium.Appium.Windows;
using OpenQA.Selenium.Remote;
internal class OutlookDemo
private static WindowsDriver<WindowsElement> session;
public void OpenOutlook() {
DesiredCapabilities appCapabilities = new DesiredCapabilities();
appCapabilities.SetCapability("app", TestContext.Parameters["AppId"]); //Getting AppId from test.runsettings.
session = new WindowsDriver<WindowsElement>(new Uri(TestContext.Parameters["sessionSingletonUrl"]), appCapabilities);// Getting sessionSingletonUrl from test.runsettings
session.SwitchTo().Window(session.WindowHandles[0]);
session.Manage().Timeouts().ImplicitWait = TimeSpan.FromSeconds(15);
session.Manage().Window.Maximize();
session.FindElementByName("Home").Click();
session.FindElementByName("Send / Receive").Click();
session.FindElementByName("View").Click();
session.FindElementByName("Help").Click();
NOTE: Before executing this script you need to select test.run settings to your current project and WinAppDriver c# should be open.

Windows Application Driver (WinAppDriver) Pros
This is an open-source tool. It uses the web driver protocol. It’s free and developed by Microsoft. WinAppDriver can integrate with selenium and Appium projects in the C# language.
Conclusion
WinAppDriver is a very easy-to-use free tool and it is the best option for Automation on Windows applications rather than a paid one, which is easy to integrate and implement with existing automation projects.
FAQs
- How do I download the window application driver(WinAppDriver)?
Configuring and Installing WinAppDriver
- Go to the windows application driver download Settings and select Developer Mode from the Developer menu.
- Download Windows Application Driver (WinAppDriver) installer.
- Download the .msi file and install it on your system.
- How do I set up WinAppDriver?
- Install and run WinAppDriver
- Run the installer on your Windows 10 computer to install and test the application you want to test on this computer.
- Enable Developer Mode in Windows Settings.
- Run WinAppDriver.exe from the installation directory (for example, C:\Program Files (x86)\Windows Application Driver).
- Does WinAppDriver support Windows 11?
WinAppDriver is a free tool (the code for WinAppDriver is not currently open source, but the FAQ says they are considering an option to make it open source). Supported on computers running WinAppDriver windows 11 (Home and Professional edition) and Microsoft Winappdriver Server 2021 operating system, it supports all Windows applications based on Windows Forms (WinForms).
- What is the difference between Winium and WinAppDriver?
Winium is an open-source test automation framework for testing WPF and WinForms applications. Like WinAppDriver, Winium is also based on the WebDriver protocol. On the other hand, Winium is not comparable to WinAppDriver in several respects.
To read more software testing-related articles and information from us here at Devstringx, kindly check out our other software testing-related blog.
Original Source:- Click Here