Agenda
In this blog, we are going to talk about the following points:-
What is Spring Boot?
- Spring Boot Application Architecture
Why Spring Boot?
- Some important Annotation of Spring boot
Let’s set up a Spring Boot application
Some Important Dependency for Spring Boot Project
Entity
Repository
Service
Controller
Helper
Constant
Router
What is Spring Boot?
Spring Boot is an open-source, microservice-based Java web framework. The Spring Boot framework creates a fully production-ready environment that is completely configurable using its prebuilt code within its codebase.
Spring Boot Application Architecture
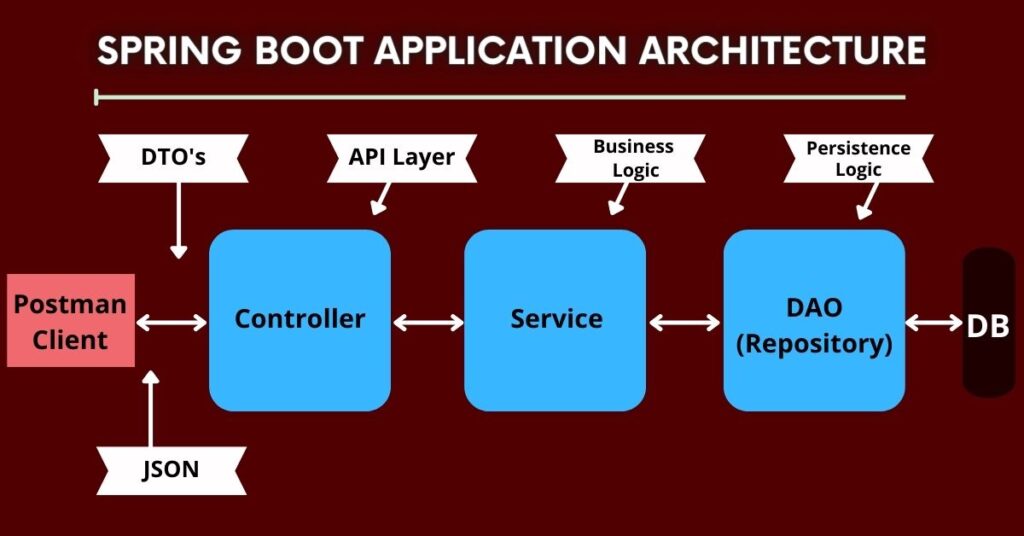
Why Should You Use Spring Boot?
- Fast and easy development of Spring-based applications
- No need for the deployment of war files
- The ability to create standalone applications
- Helping to directly embed Tomcat, Jetty, or Undertow into an application
- No need for XML configuration
- Reduced amounts of source code
- Additional out-of-the-box functionality
- Easy start
- Simple setup and management
- Large community and many training programs to facilitate the familiarization period
Some Important Annotations of Spring Boot

Spring Boot Application and Perform CRUD Operation
Step 1: Open Spring Initializr http://start.spring.io.
Step 2: Select the Spring Boot version 2.3.0.M1.
Step 2: Provide the Group name. We have provided com.demo.
Step 3: Provide the Artifact Id. We have provided spring-boot-crud-operation.
Step 5: Add the dependencies Spring Web, Spring Data JPA
Step 6: Click on the Generate button. When we click on the Generate button, it wraps the specifications in a Jar file and downloads it to the local system.
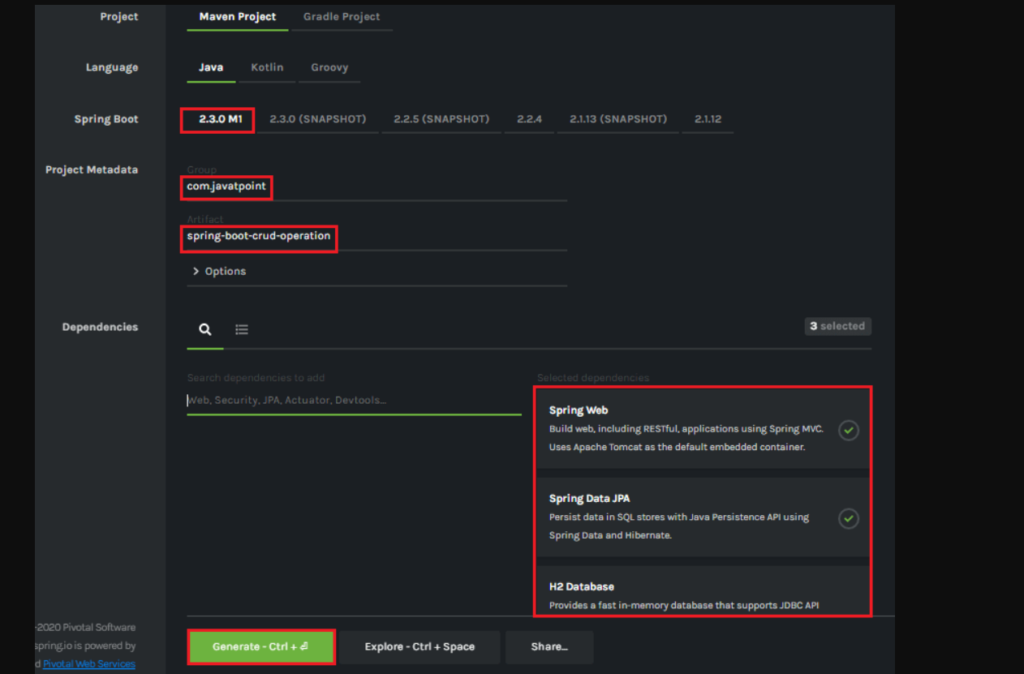
Dependencies for Spring Boot Project
// Spring boot validation
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20220320</version>
spring-boot-starter-web -> Services on Tomcat - typically REST services using Spring MVC for web layer
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
spring-boot-starter-web-services -> SOAP services
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
spring-boot-starter-jersey -> Services on Tomcat - typically REST services using Jersey implementation of JAX-RS for web layer
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jersey</artifactId>
MySQL Connector Java Maven Dependency
<artifactId>mysql-connector-java</artifactId>
//Spring boot swagger Generator
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-ui</artifactId>
//Dependency for setter getter and constructors
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
//Spring boot Jpa Dependency (ORM)
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
Create The Blow Class in Your Project

Entity Class – Book.java
package com.example.msn.Entities;
import java.sql.Timestamp;
import java.time.LocalDateTime;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@GeneratedValue(strategy = GenerationType.AUTO)
private Timestamp createdOn;
Repository Class – BookRepository.java
package com.example.msn.Repository;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import com.example.msn.Entities.Book;
public interface BookRepository extends JpaRepository<Book,Integer> {
Service Class – BookService.java
packagecom.example.msn.Services;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.example.msn.Entities.Book;
import com.example.msn.Repository.BookRepository;
private BookRepository bookRepository;
public Void deleteBook(Integer id) {
bookRepository.deleteById(id);
public List<Book> getBooks() {
List<Book> books = bookRepository.findAll();
public Book addBook(Book book1) {
Book book = bookRepository.save(book1);
public Optional<Book> findById(Integer id) {
Optional<Book> book1 = bookRepository.findById(id);
Controller – BookController.java
package com.example.msn.Controllers;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import com.example.msn.Constants.ResMesConstants;
import com.example.msn.Entities.Book;
import com.example.msn.Helpers.ApiResponse;
import com.example.msn.Requests.AddBookRequest;
import com.example.msn.Requests.EditBookRequest;
import com.example.msn.Services.BookService;
public class BookController {
private BookService bookService;
public ResponseEntity<Object> deleteBook(Integer id) {
bookService.deleteBook(id);
ApiResponse apiResponse = new ApiResponse(false, ResMesConstants.BOOK_DELETED_SUCCESSFULLY,
return new ResponseEntity<Object>(apiResponse, HttpStatus.OK);
public ResponseEntity<Object> getBooks() {
List<Book> book = bookService.getBooks();
ApiResponse apiResponse = new ApiResponse(false, ResMesConstants.RECORD_FOUND_SUCCESSFULLY,
return new ResponseEntity<Object>(apiResponse, HttpStatus.OK);
public ResponseEntity<Object> editBook(EditBookRequest editBookRequest) {
Optional<Book> book1 = bookService.findById(editBookRequest.getId());
if (book1.get() == null) {
ApiResponse apiResponse = new ApiResponse(false, ResMesConstants.SOMETHING_WENT_WRONG,
HttpStatus.PRECONDITION_FAILED,
return new ResponseEntity<Object>(apiResponse, HttpStatus.PRECONDITION_FAILED);
book1.get().setName(editBookRequest.getBookname());
book1.get().setQty(editBookRequest.getQty());
Book book = bookService.addBook(book1.get());
ApiResponse apiResponse = new ApiResponse(false, ResMesConstants.SOMETHING_WENT_WRONG,
HttpStatus.PRECONDITION_FAILED,
return new ResponseEntity<Object>(apiResponse, HttpStatus.PRECONDITION_FAILED);
ApiResponse apiResponse = new ApiResponse(false, ResMesConstants.BOOK_UPDATED_SUCCESSFULLY, HttpStatus.OK,
return new ResponseEntity<Object>(apiResponse, HttpStatus.OK);
public ResponseEntity<Object> addBook(AddBookRequest addBookRequest) {
book1.setName(addBookRequest.getBookname());
book1.setQty(addBookRequest.getQty());
Book book = bookService.addBook(book1);
ApiResponse apiResponse = new ApiResponse(false, ResMesConstants.BOOK_ADDED_SUCCESSFULLY, HttpStatus.OK,
return new ResponseEntity<Object>(apiResponse, HttpStatus.OK);
Helper – ApiResponse.java
package com.example.msn.Helpers;
import org.springframework.http.HttpStatus;
public class ApiResponse {
private HttpStatus HttpStatus;
public ApiResponse(boolean error, String message, HttpStatus httpStatus, Object data) {
this.HttpStatus = httpStatus;
Constants Constant.java
package com.example.msn.Constants;
public interface ResMesConstants {
public final String RECORD_FOUND_SUCCESSFULLY = "Record found Successfully";
public final String RECORD_NOT_FOUND = "Record not found";
public final String INVALID_TOKEN = "Invalid token";
public final String INVALID_REQUEST = "Invalid request";
public final String EMAIL_IS_ALREADY_REGISTERED = null;
public final String BOOK_DELETED_SUCCESSFULLY = null;
public final String BOOK_UPDATED_SUCCESSFULLY = null;
public final String BOOK_ADDED_SUCCESSFULLY = null;
public final String SOMETHING_WENT_WRONG = "something went wrong!";
public final String LOGIN_SUCCESSFULLY = "Login successfully";
public final String INVALID_USER_PASSWORD = "Invalid user password";
public final String PRECONDITION_FAILED = "Precondition Failed";
public final String MOBILE_NO_LENGTH_MUST_9 = "Mobile no length must >9";
public final String USER_NAME_CAN_NOT_BE_EMPTY = "User Name Can not be empty";
public final String PASSWORD_LENGTH_MUST_5 = "Password length must >5 ";
public final String MOBILE_NO_IS_ALREADY_REGISTERED = "Mobile no is already registered!";
public final String REGISTRATION_SUCCESSFULLY = "Registration successfully ";
public final String INVALID_MOBILE_NO = "Invalid Mobile No ";
Read Also- Install The Telescope In Laravel
Router openApi.java
package com.example.msn.Routes;
import javax.validation.Valid;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import com.example.msn.Controllers.BookController;
import com.example.msn.Requests.AddBookRequest;
import com.example.msn.Requests.EditBookRequest;
import com.example.msn.Requests.LoginEntityRequest;
import com.example.msn.Requests.RegistrationRequest;
private BookController bookController;
@PostMapping(path = "/addBook", consumes = "application/json")
public ResponseEntity<Object> addBook(@RequestBody AddBookRequest addBookRequest) {
return bookController.addBook(addBookRequest);
@PostMapping(path = "/editBook", consumes = "application/json")
public ResponseEntity<Object> editBook(@RequestBody EditBookRequest editBookRequest) {
return bookController.editBook(editBookRequest);
@GetMapping(path = "/getBooks")
public ResponseEntity<Object> getBooks() {
return bookController.getBooks();
@PostMapping(path = "/deleteBook/{id}")
public ResponseEntity<Object> deleteBook(@PathVariable(name = "id" ) String id) {
return bookController.deleteBook(Integer.valueOf(id));
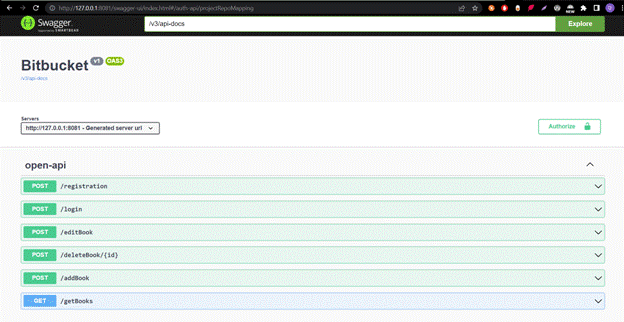
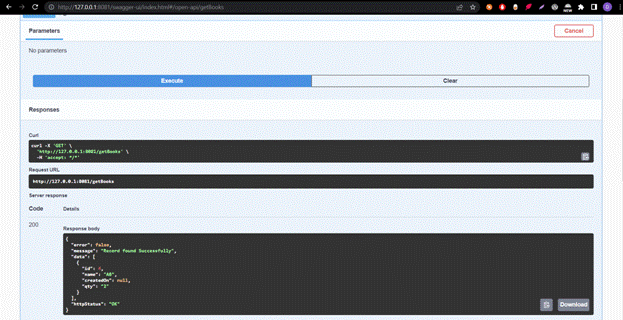
Original Source:- Click Here
No comments:
Post a Comment